可以注入的类型:Array、List、Set、Map、Properties。(虽然Map不是Collection)
BeanCollections.java文件:
package com.explause.CollectionInjectExamples;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
public class BeanCollections {
private Object[] array;
private List<?> list;
private Set<?> set;
private Map<?, ?> map;
private Properties prop;
public void setArray(Object[] array) {
this.array = array;
}
public void getArray() {
System.out.print("Array Elements :");
for (Object i : array) {
System.out.print(i);
System.out.print(", ");
}
System.out.println();
}
public void setList(List<?> list) {
this.list = list;
}
public void getList() {
System.out.println("List Elements :" + list);
}
public void setSet(Set<?> set) {
this.set = set;
}
public void getSet() {
System.out.println("Set Elements :" + set);
}
public void setMap(Map<?, ?> map) {
this.map = map;
}
public void getMap() {
System.out.println("Map Elements :" + map);
}
public void setProp(Properties prop) {
this.prop = prop;
}
public void getProp() {
System.out.println("Properties Elements :" + prop);
}
}
CollectionInjectDemo.java文件:
package com.explause.CollectionInjectExamples;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class CollectionInjectDemo {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml");
BeanCollections bc = (BeanCollections) context.getBean("beanCollections");
bc.getArray();
bc.getList();
bc.getMap();
bc.getProp();
bc.getSet();
context.close();
}
}
Beans.xml配置文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id='beanCollections' class='com.explause.CollectionInjectExamples.BeanCollections'>
<property name="array">
<array>
<value>1</value>
<value>2</value>
<value>3</value>
<value>4</value>
</array>
</property>
<property name="list">
<list>
<value>1</value>
<value>2</value>
<value>3</value>
<value>4</value>
</list>
</property>
<property name="set">
<set>
<value>one</value>
<value>two</value>
<value>three</value>
<value>three</value>
</set>
</property>
<property name="map">
<map>
<entry key='1' value='one'/>
<entry key='2' value='two'/>
<entry key='3' value='three'/>
<entry key='4' value='four'/>
</map>
</property>
<property name="prop">
<props>
<prop key="one">一</prop>
<prop key="two">二</prop>
<prop key="three">三</prop>
<prop key="four">四</prop>
</props>
</property>
</bean>
</beans>
最后的输出结果:
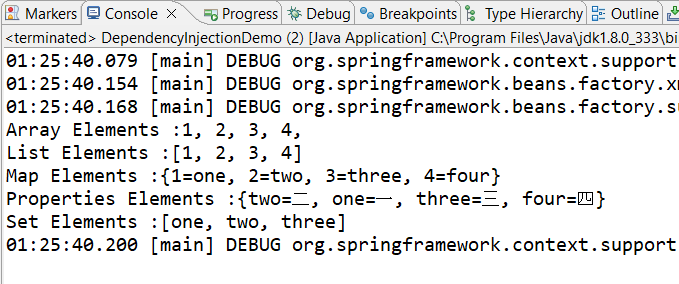
就像一般的依赖注入一样执行。只是要注意property标签里面的子标签以及它们的子标签,list和set都是value,map是entry,props是prop。
就是bean配置文件又臭又长。