bean标签的autowire(自动装配)属性可选的值有:
no | 默认,不执行自动装配 |
byName | 通过setter方法名称来确定 |
byType | 通过setter方法的参数类型确定 |
constructor | 通过构造函数参数类型确定 |
autodetect | 先constructor然后byType |
自动装配也有一些限制:
- <constructor-arg> 和 <property>会覆盖autowire的设定。
- 不可以装配原始(基本)数据类型、字符串和类。
- 很容易混淆。
自动装配是让容器将Bean内的变量(字段或属性)自动配置好的技术。
比如:在HelloWorld类里面有个变量HelloWorld2,这时候只要将HelloWorld注册成Bean并写好setter方法或者构造函数就能让容器自动去配置HelloWorld2,当然了,前提是HelloWorld2也注册为Bean。
DependencyInjectionDemo.java文件:
package com.explause.AutowireExamples;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class DependencyInjectionDemo {
public static void main(String[] args) {
ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("Beans.xml");
HelloWorld obj1 = (HelloWorld) context.getBean("helloWorld");
obj1.getMessage();
context.close();
}
}
HelloWorld.java文件:
package com.explause.AutowireExamples;
public class HelloWorld {
private HelloWorld2 helloWorld2;
public void setHelloWorld2(HelloWorld2 helloWorld2) {
this.helloWorld2 = helloWorld2;
}
public void getMessage() {
helloWorld2.getMessage();
}
}
HelloWorld2.java文件:
package com.explause.AutowireExamples;
public class HelloWorld2 {
private String message;
private String message2;
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
System.out.println("Your Message 2 : " + message2);
}
public void setMessage2(String message2){
this.message2 = message2;
}
}
以及Bean注册文件Beans.xml:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation = "http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd">
<bean id='helloWorld' class='com.explause.AutowireExamples.HelloWorld' autowire="byType"/>
<bean id='helloWorld2' class='com.explause.AutowireExamples.HelloWorld2'>
<property name="message" value='Hello World'/>
<property name="message2" value='Hello World 2'/>
</bean>
</beans>
上面id为helloWorld的bean内没有配置任何东西,只是在bean标签内添加了autowire属性,示例配置成byType。运行main函数(方法)之后并不会报错,而是显示helloWorld2的内容:
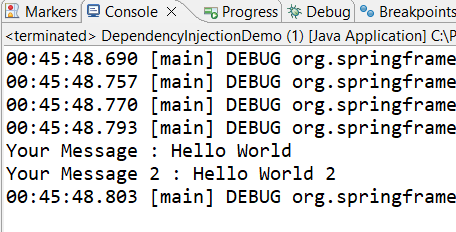