就是使用注解进行配置,不需要Beans.xml文件。在Spring Boot中很常用。常见的注解:
@Required | 作用于setter方法,指定属性必须存在于Beans.xml(已废弃) |
@Autowired | 控制自动装配 |
@Qualifier | 有多个匹配条件的bean时限定一个使用 |
@Configuration | 作用于class告诉IoC容器这是bean定义源 |
@Bean | 作用于方法,告诉Spring它返回一个对象,将被注册为bean |
@Import | 允许从另外一个class里面导入bean |
@Scope | 定义bean的作用域 |
然后创建3个Bean的定义,HelloWorld.java:
package com.explause.AnnotationConfigExamples;
public class HelloWorld {
private String message;
private HelloWorld2 hw2;
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
hw2.getMessage();
}
public HelloWorld(HelloWorld2 hw2) {
this.hw2 = hw2;
}
public void initMethod() {
System.out.println("This is the initMethod in HelloWorld.");
}
public void destroyMethod() {
System.out.println("This is the destroyMethod in HelloWorld.");
}
}
HelloWorld2.java:
package com.explause.AnnotationConfigExamples;
public class HelloWorld2 {
private String message;
private String message2;
public void setMessage(String message){
this.message = message;
}
public void getMessage(){
System.out.println("Your Message : " + message);
System.out.println("Your Message 2: " + message2);
}
public void setMessage2(String message2){
this.message2 = message2;
}
public void initMethod() {
System.out.println("This is the initMethod in HelloWorld2.");
}
public void destroyMethod() {
System.out.println("This is the destroyMethod in HelloWorld2.");
}
}
AutowiredBean.java:
package com.explause.AnnotationConfigExamples;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
public class AutowiredBean {
@Autowired
private HelloWorld hw;
@Autowired
@Qualifier("hw3")
private HelloWorld2 hw2;
public void setHelloWorld(HelloWorld hw) {
this.hw = hw;
}
public void setHelloWorld2(HelloWorld2 hw2) {
this.hw2 = hw2;
}
public HelloWorld getHelloWorld() {
return hw;
}
public HelloWorld2 getHelloWorld2() {
return hw2;
}
public void getMessages() {
System.out.println("Inside the AutowiredBean.");
hw.getMessage();
hw2.getMessage();
}
}
@Qualifier(“hw3”)注解的意思是在容器内选取名字叫hw3的Bean,下面会创建两个类型为HelloWorld2的Bean。
Bean配置文件,BeanConfig.java:
package com.explause.AnnotationConfigExamples;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import org.springframework.context.annotation.Scope;
@Configuration
@Import({ AnotherBeanConfig.class })
public class BeanConfig {
@Scope("singleton")
@Bean(initMethod="initMethod", destroyMethod="destroyMethod")
public HelloWorld hw(HelloWorld2 hw2) {
HelloWorld hw = new HelloWorld(hw2);
hw.setMessage("message in hw");
return hw;
}
@Bean(initMethod="initMethod", destroyMethod="destroyMethod")
public HelloWorld2 hw2() {
HelloWorld2 hw2 = new HelloWorld2();
hw2.setMessage("message1 in HelloWorld2");
hw2.setMessage2("message2 in HelloWorld2");
return hw2;
}
@Bean
public HelloWorld2 hw3() {
HelloWorld2 hw2 = new HelloWorld2();
hw2.setMessage("message1 in HelloWorld3");
hw2.setMessage2("message2 in HelloWorld3");
return hw2;
}
}
上面的代码中,决定一个Bean名字的是函数(方法)的名字,比如hw、hw2和hw3。
@Bean注解作用的函数(方法)返回的对象就是会被添加到容器内的Bean。
生成Bean对象的函数可以有参数,Spring框架会自动解析依赖的对象,但是这些参数需要是Bean,
这也就是所谓IoC(Inverse of Control)的意义,对象全都由容器控制。
再创建一个Bean配置文件,AnotherBeanConfig.java:
package com.explause.AnnotationConfigExamples;
import org.springframework.context.annotation.Bean;
public class AnotherBeanConfig {
@Bean
public AutowiredBean autowiredBean() {
return new AutowiredBean();
}
}
很简单,创建一个Bean,名字叫hw3。
最后创建main函数(方法),CollectionInjectDemo .java文件:
package com.explause.AnnotationConfigExamples;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class CollectionInjectDemo {
public static void main(String[] args) {
AnnotationConfigApplicationContext context =
new AnnotationConfigApplicationContext("com.explause.AnnotationConfigExamples");
AutowiredBean ab = context.getBean(AutowiredBean.class);
ab.getMessages();
context.close();
}
}
容器(ApplicationContext)的类型由ClassPathXmlApplicationContext变为AnnotationConfigApplicationContext了,这个类很重要,会用在Spring Boot里面。
它的构造函数参数可以指定自动扫描的包路径,然后自动处理包内类文件的注解。
最终的输出结果:
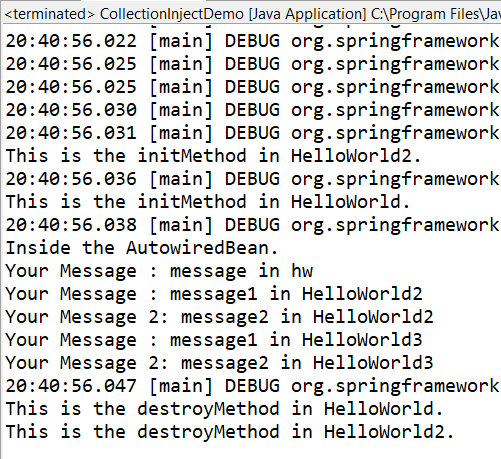
名为hw的函数(方法)在名字在hw2之前,但是因为创建这个Bean需要用到类型为HelloWorld2的Bean,
正好容器(ApplicationContext)内有这个Bean的定义,所以要先初始化它,也就有了HelloWorld2在HelloWorld之前初始化。自动装配(@Autowired)的变量,容器会让它指向容器内的Bean对象。