SpringMVC处理POST请求时可以将收到的参数自动转换成POJO类型的类对象,所以只要定义一个POJO类,就能很简单的进行处理。
然后一顿复制粘贴:
POJO.java:
package com.explause.main;
public class POJO {
private String msg1;
private String msg2;
public void setMsg1(String msg1) {
this.msg1 = msg1;
}
public String getMsg1() {
return this.msg1;
}
public void setMsg2(String msg2) {
this.msg2 = msg2;
}
public String getMsg2() {
return this.msg2;
}
}
AppController.java:
package com.explause.main;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.servlet.ModelAndView;
@Controller
public class AppController {
private ModelAndView md = new ModelAndView();
@GetMapping("/")
public ModelAndView getController() {
md.setViewName("get");
return md;
}
@PostMapping("/")
public ModelAndView postController(POJO getinfo) {
md.setViewName("post");
md.addObject("messages", getinfo);
return md;
}
}
web.xml:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" id="WebApp_ID" version="4.0">
<display-name>POSTDemo</display-name>
<servlet>
<servlet-name>App</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>App</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
App-servlet.xml:
<beans xmlns = "http://www.springframework.org/schema/beans"
xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance"
xmlns:context = "http://www.springframework.org/schema/context"
xmlns:aop = "http://www.springframework.org/schema/aop"
xmlns:mvc = "http://www.springframework.org/schema/mvc"
xsi:schemaLocation = "http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-3.0.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd">
<context:component-scan base-package="com.explause.main"/>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/"/>
<property name="suffix" value=".jsp"/>
</bean>
</beans>
get.jsp:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Get some messages</title>
</head>
<body>
<form method="POST" action="">
<label>Give some string:</label>
<input name="msg1"/>
<label>Give another string:</label>
<input name="msg2"/>
<input type="submit" value="提交"/>
</form>
</body>
</html>
post.jsp:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Submitted messages</title>
</head>
<body>
<p>The first message:</p>
<p>${messages.msg1}
<p>The second message:</p>
<p>${messages.msg2}
</body>
</html>
最终的项目目录结构:
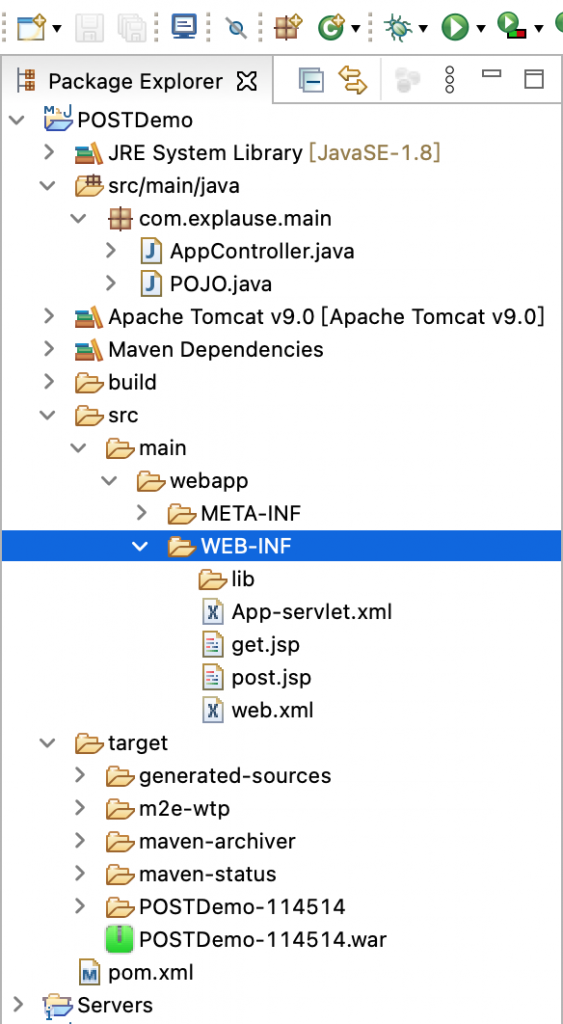
注意,和web相关的代码,根目录是webapp文件夹,App-servlet.xml文件中的prefix属性就是从webapp文件夹开始的。当然,可以在这个文件夹下自定义文件存放位置。
运行结果:

点击提交后:
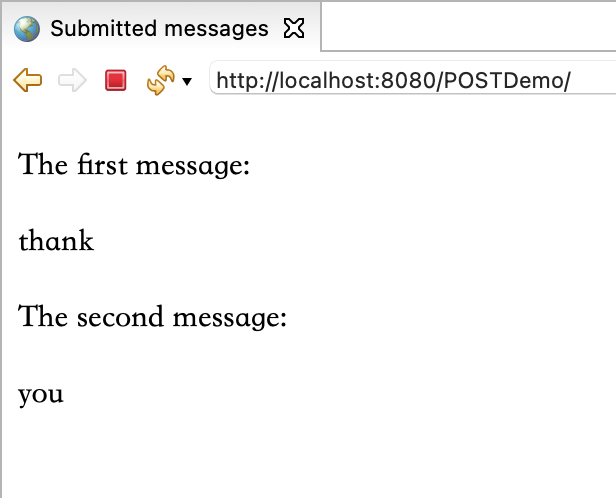
分析一下:
Controller可以返回String对象,也可以返回ModelAndView对象。ModelAndView类可以接受一个 viewName参数,指定某个View(jsp文件或别的,根据App-servlet.xml内prefix和suffix参数决定)。处理POST请求的控制器决定处理了POST请求后返回的View,因为View是jsp,需要和Model相相加才能生成最后的HTML。ModelAndView类的addObject方法可以为View设置Model它的第一个参数是Model名,第二个参数是Model值。Model实际上是一个Map数据类型。在jsp文件里可以像处理普通java代码一样使用它。那是jsp相关的知识了。